Many of you how read my post about the security proxy concept in FileNet P8 (http://justecm.de/2020/02/using-security-proxy-for-dynamic-access-control-in-filenet-p8/) asked me how to set the security proxy property values for existing documents in a bulk job without coding a external java program. In this post I will show you how to to this with a custom java script sweep action for property templates of data type string an object.
At first you need to create a new custom sweep action in the sweep management of you object store.
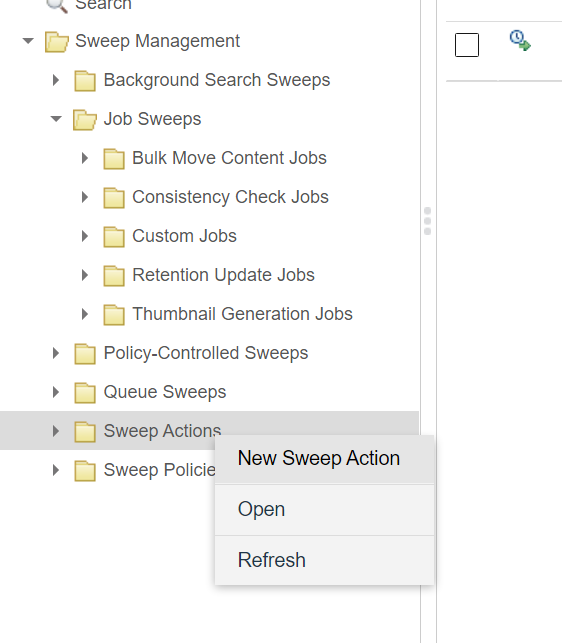
Give the action a name and select “JavaScript” as the action type.
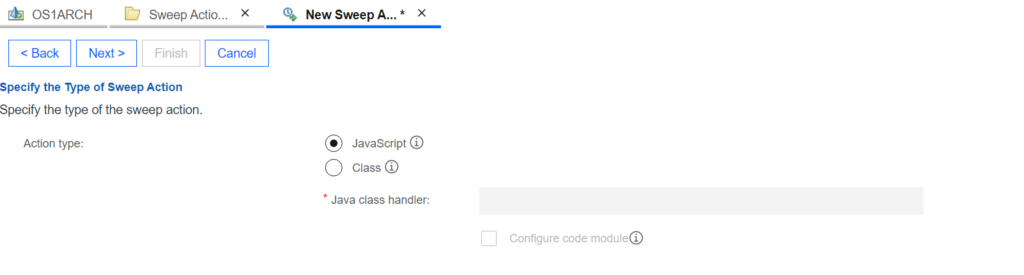
Enter the following Javascript code in the appropriate field and finish the sweep action creation.
importPackage(Packages.com.filenet.api.core);
importPackage(Packages.com.filenet.api.constants);
importPackage(Packages.com.filenet.api.exception);
importPackage(Packages.com.filenet.api.sweep);
importPackage(Packages.com.filenet.api.engine);
importPackage(Packages.com.filenet.api.property);
importPackage(Packages.com.filenet.api.util);
// Implement for custom job and queue sweeps.
function onSweep (sweepObject, sweepItems)
{
var hcc = HandlerCallContext.getInstance();
hcc.traceDetail("Entering CustomSweepHandler.onSweep");
hcc.traceDetail("sweepObject = " + sweepObject.getProperties().getIdValue(PropertyNames.ID) + "sweepItems.length = " + sweepItems.length);
// Iterate the sweepItems and change the class.
ii = 0;
for (ii = 0; ii < sweepItems.length; ii++)
{
// At the top of your loop, always check to make sure
// that the server is not shutting down.
// If it is, clean up and return control to the server.
if (hcc != null && hcc.isShuttingDown())
{
throw new EngineRuntimeException(ExceptionCode.E_BACKGROUND_TASK_TERMINATED, this.constructor.name + " is terminating prematurely because the server is shutting down");
}
var item = sweepItems[ii].getTarget();
var msg = "sweepItems[" + ii + "]= " + item.getProperties().getIdValue("ID");
hcc.traceDetail(msg);
try
{
var CEObject = com.filenet.api.core.Document (item);
var os = sweepObject.getObjectStore()
var objectId = com.filenet.api.util.Id("{5BE7719F-9B56-4183-8F5A-7D3C514FA765}");
var co = Factory.CustomObject.fetchInstance(os, objectId, null);
CEObject.getProperties().putObjectValue("SecurityTemplate", co);
CEObject.save(com.filenet.api.constants.RefreshMode.NO_REFRESH);
// Set outcome to PROCESSED if item processed successfully.
sweepItems[ii].setOutcome(SweepItemOutcome.PROCESSED,
"item processed by " + this.constructor.name);
}
// Set failure status on objects that fail to process.
catch (ioe)
{
sweepItems[ii].setOutcome(SweepItemOutcome.FAILED, "CustomSweepHandler: " +
ioe.rhinoException.getMessage());
}
}
hcc.traceDetail("Exiting CustomSweepHandler.onSweep");
}
/*
* Called automatically when the handler is invoked by a custom sweep job
* or sweep policy. Specify properties required by the handler, if any.
* If you return an empty array, then all properties are fetched.
*/
function getRequiredProperties()
{
var pnames = ['Id'];
return pnames.toString();
}
/* Implement for custom sweep policies.
* This method is not implemented because this is an example of a custom sweep job.
*/
function onPolicySweep (sweepObject, policyObject, sweepItems)
{}
The only value that needs to be adapted to your environment is in line 37 the GUID of the security proxy object that you want to pass to the documents via the sweep job and in line 39 the symbolic name of the property template in your document class.
var objectId = com.filenet.api.util.Id(“{5BE7719F-9B56-4183-8F5A-7D3C514FA765}“);
CEObject.getProperties().putObjectValue(“SecurityTemplate“, co);
So if you need to set different object values to the property templates I would recommend to create also different sweep actions. It make it afterwards a little bit more handy.
Now you can create the custom sweep job to set the object property values for all you existing documents.
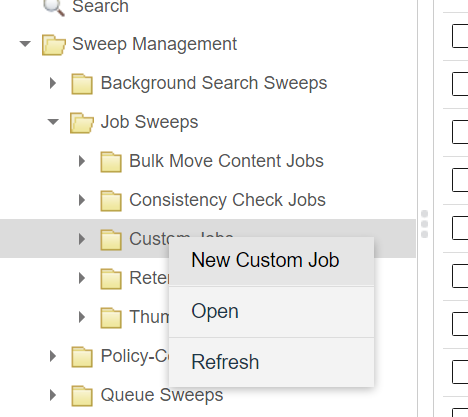
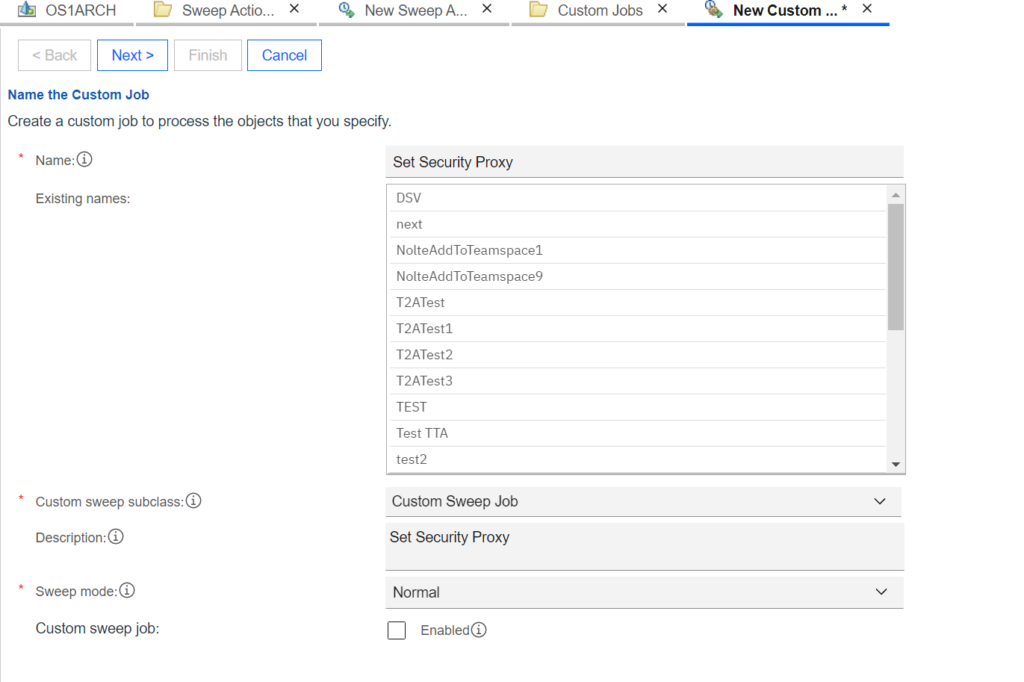
Select the target class on which you want to run the sweep and select our previously created custom sweep action.
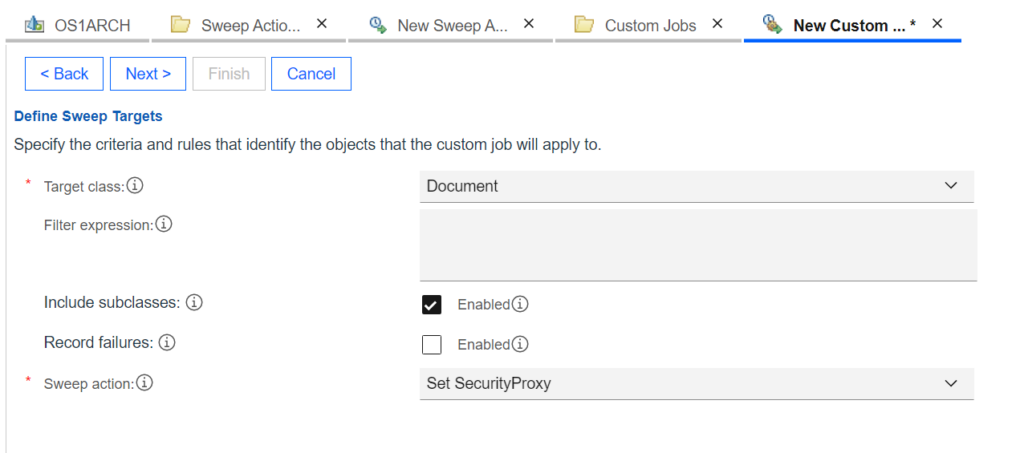
Finish the creation wizard an activate you sweep job.
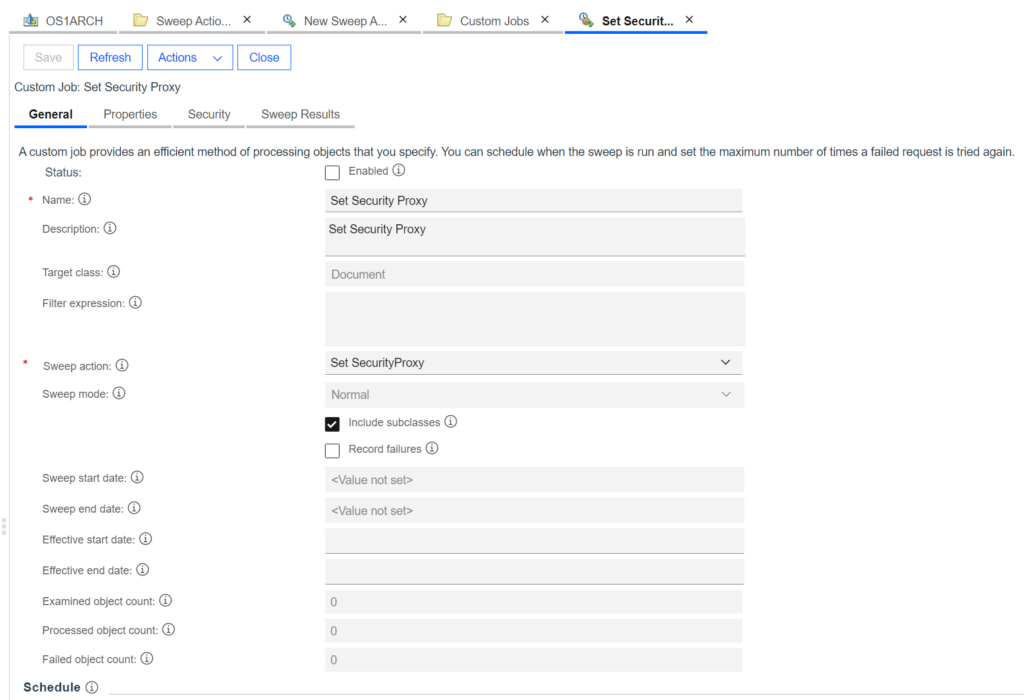
That’s it now you can update the property values with bulk processing. Now I want to share with you also the Java Script code to update a string property value.
importPackage(Packages.com.filenet.api.core);
importPackage(Packages.com.filenet.api.constants);
importPackage(Packages.com.filenet.api.exception);
importPackage(Packages.com.filenet.api.sweep);
importPackage(Packages.com.filenet.api.engine);
// Implement for custom job and queue sweeps.
function onSweep (sweepObject, sweepItems)
{
var hcc = HandlerCallContext.getInstance();
hcc.traceDetail("Entering CustomSweepHandler.onSweep");
hcc.traceDetail("sweepObject = " + sweepObject.getProperties().getIdValue(PropertyNames.ID) + "sweepItems.length = " + sweepItems.length);
// Iterate the sweepItems and change the class.
ii = 0;
for (ii = 0; ii < sweepItems.length; ii++)
{
// At the top of your loop, always check to make sure
// that the server is not shutting down.
// If it is, clean up and return control to the server.
if (hcc != null && hcc.isShuttingDown())
{
throw new EngineRuntimeException(ExceptionCode.E_BACKGROUND_TASK_TERMINATED, this.constructor.name + " is terminating prematurely because the server is shutting down");
}
var item = sweepItems[ii].getTarget();
var msg = "sweepItems[" + ii + "]= " + item.getProperties().getIdValue("ID");
hcc.traceDetail(msg);
try
{
var CEObject = com.filenet.api.core.Document (item);
CEObject.getProperties().putValue("DocumentTitle","This is a custom sweep action");
CEObject.save(com.filenet.api.constants.RefreshMode.NO_REFRESH);
// Set outcome to PROCESSED if item processed successfully.
sweepItems[ii].setOutcome(SweepItemOutcome.PROCESSED,
"item processed by " + this.constructor.name);
}
// Set failure status on objects that fail to process.
catch (ioe)
{
sweepItems[ii].setOutcome(SweepItemOutcome.FAILED, "CustomSweepHandler: " +
ioe.rhinoException.getMessage());
}
}
hcc.traceDetail("Exiting CustomSweepHandler.onSweep");
}
/*
* Called automatically when the handler is invoked by a custom sweep job
* or sweep policy. Specify properties required by the handler, if any.
* If you return an empty array, then all properties are fetched.
*/
function getRequiredProperties()
{
var pnames = ['Id'];
return pnames.toString();
}
/* Implement for custom sweep policies.
* This method is not implemented because this is an example of a custom sweep job.
*/
function onPolicySweep (sweepObject, policyObject, sweepItems)
{}
To use this in you environment you just need to set up the property templates symbolic name an the string you want to pass in code line 32. The rest is straight forward and you can work around the previous guide above.
Have fun with the sweeps :-).
Over and out!
Hey there just wanted to give you a quick heads up and let you know
a few of the pictures aren’t loading properly.
I’m not sure why but I think its a linking issue.
I’ve tried it in two different internet browsers and both show the same outcome.
If you would like to grow your familiarity just
keep visiting this site and be updated with the most up-to-date information posted here.